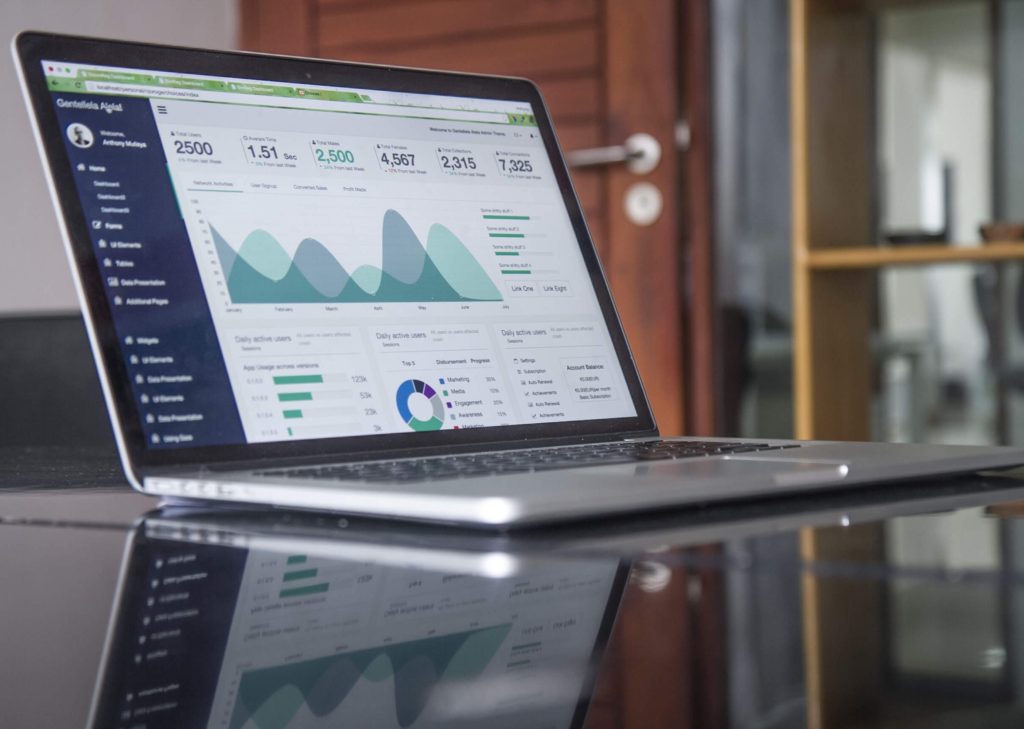
どのグラフも、あくまでサンプルです。
折れ線グラフ
まずは、折れ線グラフ。
毎月末に英語のテストがあったと仮定して見てみてください。
<script>
var ctx = document.getElementById(“lineGraph”);
var myLineChart = new Chart(ctx, {
type: ‘line’,
data: {
labels: [‘4月’, ‘5月’, ‘6月’, ‘7月’, ‘8月’, ‘9月’, ’10月’, ’11月’],
datasets: [
{
label: ‘A君’,
data: [35, 42, 65, 78, 55, 61, 80, 69],
borderColor: “red”,
backgroundColor: “rgba(0,0,0,0)”
},
{
label: ‘B君’,
data: [44, 48, 42, 37, 55, 68, 82, 90],
borderColor: “blue”,
backgroundColor: “rgba(0,0,0,0)”
},
],
},
options: {
title: {
display: true,
text: ‘英語のテストの点数推移’
},
scales: {
yAxes: [{
ticks: {
suggestedMax: 100,
suggestedMin: 0,
stepSize: 10,
callback: function(value, index, values){
return value + ‘点’
}
}
}]
},
}
});
</script>
棒グラフ
次に棒グラフ。
折れ線グラフのデータを棒グラフで表示してみましょう。
変更したポイントは、以下の3点
・IDを変えました
・typeをbarにしました
・border-colorを削除し、その色をbackground-colorに設定しました
<script>
var ctx = document.getElementById(“barGraph”);
var myLineChart = new Chart(ctx, {
type: ‘bar’,
data: {
labels: [‘4月’, ‘5月’, ‘6月’, ‘7月’, ‘8月’, ‘9月’, ’10月’, ’11月’],
datasets: [
{
label: ‘A君’,
data: [35, 42, 65, 78, 55, 61, 80, 69],
backgroundColor: “red”
},
{
label: ‘B君’,
data: [44, 48, 42, 37, 55, 68, 82, 90],
backgroundColor: “blue”
},
],
},
options: {
title: {
display: true,
text: ‘英語のテストの点数推移’
},
scales: {
yAxes: [{
ticks: {
suggestedMax: 100,
suggestedMin: 0,
stepSize: 10,
callback: function(value, index, values){
return value + ‘点’
}
}
}]
},
}
});
</script>
棒グラフ(横)
↑で作った棒グラフを横向きにしてみましょう。
typeは「horizontalBar」を使用します。
<script>
var ctx = document.getElementById(“barGraph2”);
var myLineChart = new Chart(ctx, {
type: ‘horizontalBar’,
data: {
labels: [‘4月’, ‘5月’, ‘6月’, ‘7月’, ‘8月’, ‘9月’, ’10月’, ’11月’],
datasets: [
{
label: ‘A君’,
data: [35, 42, 65, 78, 55, 61, 80, 69],
backgroundColor: “red”
},
{
label: ‘B君’,
data: [44, 48, 42, 37, 55, 68, 82, 90],
backgroundColor: “blue”
},
],
},
options: {
title: {
display: true,
text: ‘英語のテストの点数推移’
},
scales: {
xAxes: [{
ticks: {
suggestedMax: 100,
suggestedMin: 0,
stepSize: 10,
callback: function(value, index, values){
return value + ‘点’
}
}
}]
},
}
});
</script>
棒グラフ+折れ線グラフ
↑で作成した折れ線グラフに棒グラフで平均点を追加してみましょう。
<script>
var ctx = document.getElementById(“lineBarGraph”);
var myLineChart = new Chart(ctx, {
type: ‘bar’,
data: {
labels: [‘4月’, ‘5月’, ‘6月’, ‘7月’, ‘8月’, ‘9月’, ’10月’, ’11月’],
datasets: [
{
label: ‘A君’,
type: ‘line’,
data: [35, 42, 65, 78, 55, 61, 80, 69],
borderColor: “red”,
backgroundColor: “rgba(0,0,0,0)”
},
{
label: ‘B君’,
type: ‘line’,
data: [44, 48, 42, 37, 55, 68, 82, 90],
borderColor: “blue”,
backgroundColor: “rgba(0,0,0,0)”
},
{
label: ‘平均点’,
type: ‘bar’,
data: [60, 66, 58, 62, 70, 64, 66, 71],
backgroundColor: “rgb(222,222,222)”
}
],
},
options: {
title: {
display: true,
text: ‘英語のテストの点数推移’
},
scales: {
yAxes: [{
ticks: {
suggestedMax: 100,
suggestedMin: 0,
stepSize: 10,
callback: function(value, index, values){
return value + ‘点’
}
}
}]
},
}
});
</script>
円グラフ
次は円グラフです。
ちょっと同じ例題だと円グラフにマッチしないので、シチュエーションを変えてみます。
上の例題がテストだったので、クラスの得意教科の割合にしてみましょう。
<script>
var ctx = document.getElementById(“pieGraph”);
var myLineChart = new Chart(ctx, {
type: ‘pie’,
data: {
labels: [‘英語’, ‘国語’, ‘数学’, ‘理科’, ‘社会’],
datasets: [
{
data: [6,8,5,4,7],
backgroundColor: [“red”, “blue”, “green”, “yellow”, “purple”]
},
],
},
options: {
title: {
display: true,
text: ‘クラス内の得意教科’
},
}
});
</script>
ドーナツチャート
上で作った円グラフのチャートをドーナツ型にしてみます。
個人的にはドーナツの方がおしゃれに見えますね。
<script>
var ctx = document.getElementById(“doughnutGraph”);
var myLineChart = new Chart(ctx, {
type: ‘doughnut’,
data: {
labels: [‘英語’, ‘国語’, ‘数学’, ‘理科’, ‘社会’],
datasets: [
{
data: [6,8,5,4,7],
backgroundColor: [“red”, “blue”, “green”, “yellow”, “purple”]
},
],
},
options: {
title: {
display: true,
text: ‘クラス内の得意教科’
},
}
});
</script>
鶏頭図
鶏の頭みたいなグラフ。
上の円、ドーナツをそのまま鶏頭図にしてみる。
<script>
var ctx = document.getElementById(“polarAreaGraph”);
var myLineChart = new Chart(ctx, {
type: ‘polarArea’,
data: {
labels: [‘英語’, ‘国語’, ‘数学’, ‘理科’, ‘社会’],
datasets: [
{
data: [6,8,5,4,7],
backgroundColor: [“red”, “blue”, “green”, “yellow”, “purple”]
},
],
},
options: {
title: {
display: true,
text: ‘クラス内の得意教科’
},
}
});
</script>
レーダーチャート
<script>
var ctx = document.getElementById(“radarGraph”);
var myLineChart = new Chart(ctx, {
type: ‘radar’,
data: {
labels: [‘英語’, ‘国語’, ‘数学’, ‘理科’, ‘社会’],
datasets: [{
label: ‘A君’,
data: [80, 72, 91, 86, 67],
backgroundColor: ‘RGBA(225, 0, 0, 0.5)’,
borderColor: ‘RGBA(225, 0, 0, 1)’,
borderWidth: 1,
pointBackgroundColor: ‘RGB(255, 0, 0)’
}, {
label: ‘B君’,
data: [84, 98, 57, 62, 79],
backgroundColor: ‘RGBA(0, 255, 0, 0.5)’,
borderColor: ‘RGBA(0, 255, 0, 1)’,
borderWidth: 1,
pointBackgroundColor: ‘RGB(0, 255, 0)’
}]
},
options: {
title: {
display: true,
text: ‘5教科比較’
},
scale:{
ticks:{
suggestedMin: 0,
suggestedMax: 100,
stepSize: 10,
callback: function(value, index, values){
return value + ‘点’
}
}
}
}
});
</script>
バブルチャート
typeはbubble